Circle Formulas Tutorial
This tutorial is about C structure and style. It is designed to provide information on how to effectively use indentation, comments, and other elements that make your C code more readable. Except that, the way of how to use basic data types like float is illustrated by the example of circle formulas. For the first time, we introduce the const keyword, which is #define.
/*
This is a tutorial program designed by PRL.
Author: Mike Min
Email: prlatlab@gmail.com
Data: 11/Feb/2017
*/ //(multiple line comment)
#include <stdio.h>
// blank line
#define pi 3.1415926
int main(int argc, char *argv[])
{
printf("Hello, welcome to Prl tutorial!\n");
int Prl_int, a_int, c_int; // define int variables (single line comment)
float Prl_flt, a_flt, c_flt; // define float variables
scanf("%d",&Prl_int); // input one int integer
printf("Prl_int = %d\n",Prl_int); // print the input value on the screen
a_int = pi * Prl_int * Prl_int; // calculate the area
printf("The area of a circle whose radius is %d = %d\n",Prl_int,a_int); // print the value on the screen
c_int = 2 * pi * Prl_int; // calculate the circumference
printf("The circumference of a circle whose radius is %d = %d\n",Prl_int,c_int); // print the value on the screen
scanf("%f",&Prl_flt); // input one float number
printf("Prl_flt = %f\n",Prl_flt); // print the input value on the screen
a_flt = pi * Prl_flt * Prl_flt; // calculate the area
printf("The area of a circle whose radius is %f = %f\n",Prl_flt,a_flt); // print the value on the screen
c_flt = 2 * pi * Prl_flt; // calculate the circumference
printf("The circumference of a circle whose radius is %f = %f\n",Prl_flt,c_flt); // print the value on the screen
return 0; // return 0
}

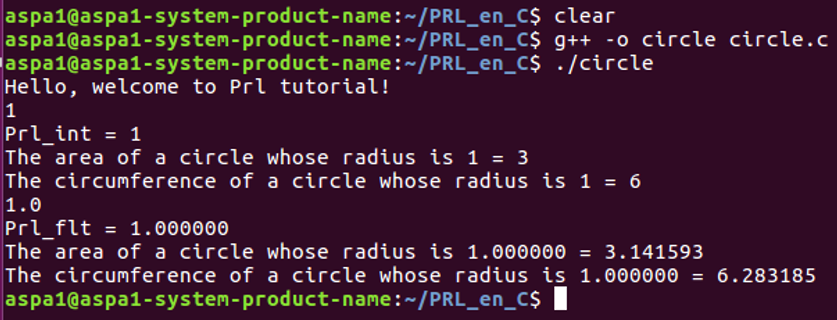