Magic Letter Tutorial
ASCII stands for American Standard Code for information interchange. Computers can only understand numbers, so an ASCII code is the numerical representation of a character such as 'a'. This tutorial is designed to describe the methods of how to use the table for the first reason. Besides, the operators of ++ and --, the relation operators like <=, the cast operators the , the variable types of char, string, bool etc are shown here.

#include <stdio.h>
int main(int argc, char *argv[])
{
char char1, charLower, charUpper,charBef, charAft; /* define char characters */
bool IsUpper, IsLower; /*define Bool variables*/
printf("Hello, welcome to Prl tutorial!\n");
scanf("%c",&char1); // input one letter
printf(" char1 = %c\n",char1); // print the input letter on the screen
IsLower = (97 <= char1 <= 122);
IsUpper = (65 <= char1 <= 90);
if ( IsLower)
{
charUpper = char1 - 32;
printf(" the upper case of %c is %c.\n",char1, charUpper);
charBef = char1 - 1;
charAft = char1 + 1;
if (char1 == 97)
{
printf(" %c is the first letter.\n",char1);
printf(" the letter after %c is %c\n",char1, charAft);
}else if (char1 == 122)
{
printf(" %c is the last letter.\n",char1);
printf(" the letter before %c is %c\n",char1, charBef);
}else
{
printf(" the letter before %c is %c\n",char1, charBef);
printf(" the letter after %c is %c\n",char1, charAft);
}
}
return 0; // return 0
}
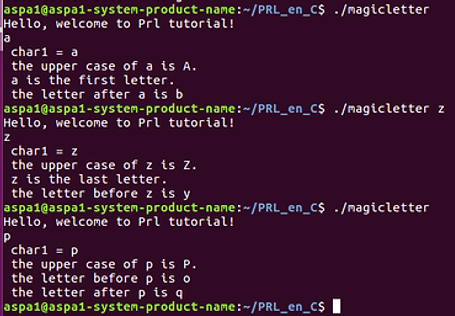